惯性#
介绍#
VEX AIM 编程机器人的惯性传感器内置一个用于测量旋转运动的三轴陀螺仪和一个用于检测运动变化的三轴加速度计。这些传感器使机器人能够追踪其方向、航向和加速度。以下是所有可用方法的列表:
方向——获取机器人的航向和角度。
get_rotation – 返回机器人自启动以来转动的距离。
get_heading – 返回当前航向(0 到 359.99°)。
get_yaw - 返回偏航角(-180 到 180°)。
get_roll - 返回滚动角度(-180 到 180°)。
get_pitch - 返回俯仰角(-90 到 90°)。
reset_rotation – 将旋转值重置为零。
reset_heading – 将当前航向设置为零。
set_heading – 将航向设置为特定值。
碰撞——检测碰撞。
运动——测量加速度和转弯速度。
get_acceleration – 返回特定方向的加速度。
get_turn_rate – 返回转弯速率(以度/秒为单位)。
校准——管理传感器校准。
calibrate – 校准惯性传感器。
is_calibrating – 返回传感器当前是否正在校准。
方向#
get_rotation#
get_rotation
以浮点数形式返回机器人的净旋转度数。
用法:
robot.inertial.get_rotation()
参数 |
描述 |
---|---|
该方法没有参数。 |
# Display the robot's rotation as it rotates
while True:
robot.screen.clear_screen()
robot.screen.set_cursor(1, 1)
robot.screen.print(f"Rotation: {robot.inertial.get_rotation():.2f}")
wait(50, MSEC)
get_heading#
get_heading
以浮点数形式返回机器人的航向角,范围在 0 到 359.99 度之间。
用法:
robot.inertial.get_heading()
参数 |
描述 |
---|---|
该方法没有参数。 |
# Turn right until the heading reaches 90 degrees
robot.turn(RIGHT)
while robot.inertial.get_heading() < 90:
wait(50, MSEC)
robot.stop_all_movement()
# Display the robot's heading as it is rotated by hand
while True:
robot.screen.clear_screen()
robot.screen.set_cursor(1, 1)
robot.screen.print(f"Heading: {robot.inertial.get_heading()} degrees")
wait(50, MSEC)
get_yaw#
get_yaw
以浮点数形式返回机器人在 -180.00 到 180.00 度范围内的偏航角。
下图使用箭头显示偏航的正旋转方向。
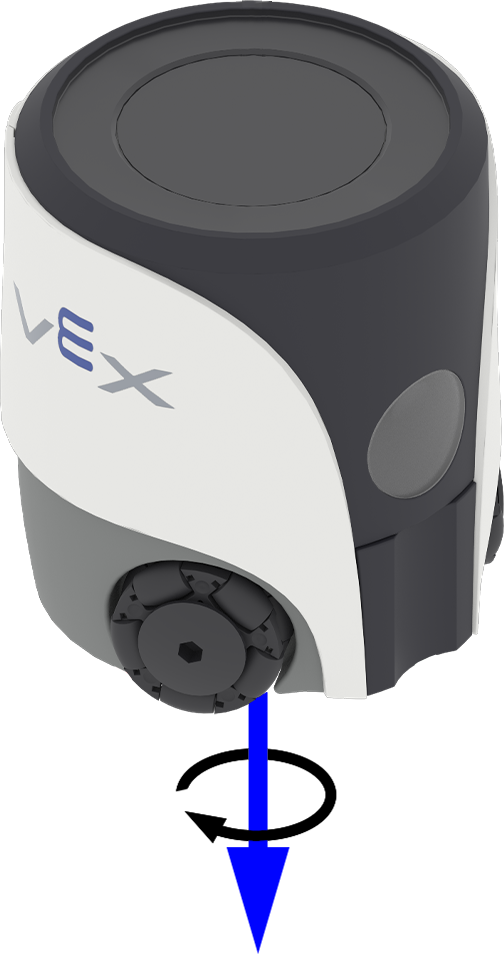
用法:
robot.inertial.get_yaw()
参数 |
描述 |
---|---|
该方法没有参数。 |
# Display the robot's yaw angle as it is rotated by hand
while True:
robot.screen.clear_screen()
robot.screen.set_cursor(1, 1)
robot.screen.print(robot.inertial.get_yaw())
wait(50, MSEC)
get_roll#
get_roll
以浮点数形式返回机器人在 -180.00 到 180.00 度范围内的滚动角度。
下图使用箭头表示滚动的正向旋转方向。
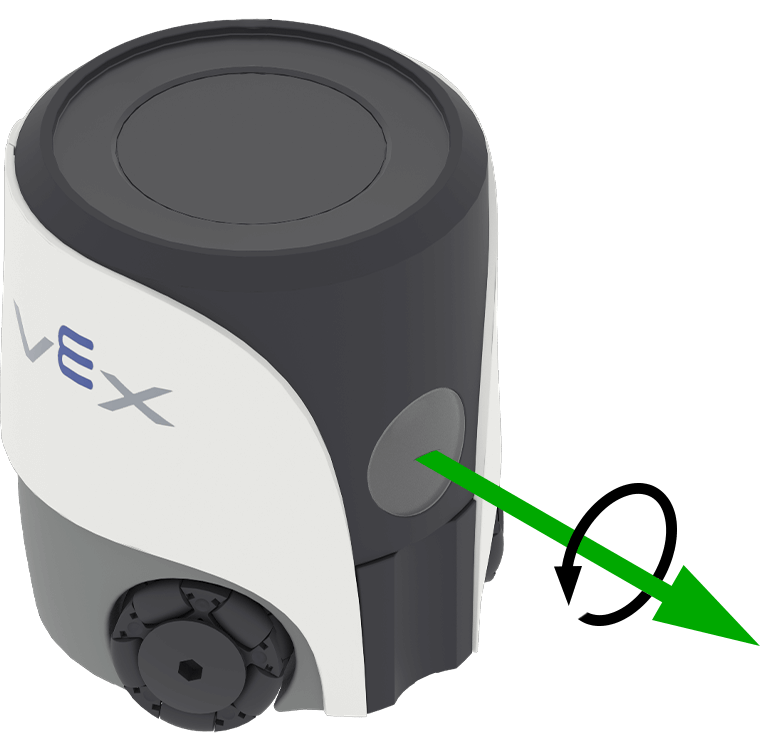
用法:
robot.inertial.get_roll()
参数 |
描述 |
---|---|
该方法没有参数。 |
# Display the robot's roll angle as it is tilted by hand
while True:
robot.screen.clear_screen()
robot.screen.set_cursor(1, 1)
robot.screen.print(robot.inertial.get_roll())
wait(50, MSEC)
get_pitch#
get_pitch
以浮点数形式返回机器人的俯仰角,范围在 -90.00 到 90.00 度之间。
下图使用箭头表示俯仰正向旋转的方向。
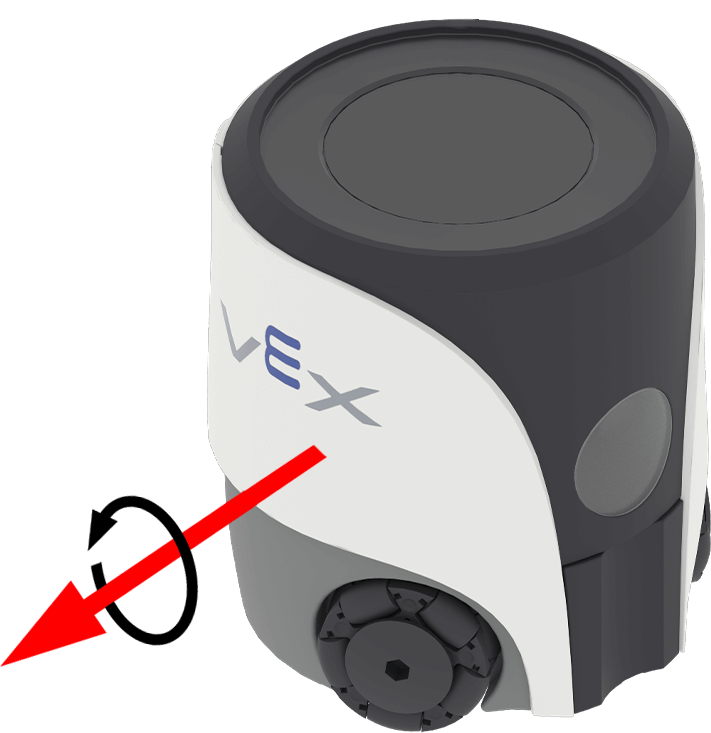
用法:
robot.inertial.get_pitch()
参数 |
描述 |
---|---|
该方法没有参数。 |
# Display the robot's pitch angle as it is tilted by hand
while True:
robot.screen.clear_screen()
robot.screen.set_cursor(1, 1)
robot.screen.print(robot.inertial.get_pitch())
wait(50, MSEC)
reset_rotation#
reset_rotation
将机器人的陀螺仪旋转值重置为 0 度。
用法:
robot.inertial.reset_rotation()
参数 |
描述 |
---|---|
该方法没有参数。 |
# Reset the robot's rotation if it exceeds 180 degrees
while True:
if robot.inertial.get_rotation() >= 180:
robot.inertial.reset_rotation()
robot.screen.clear_screen()
robot.screen.set_cursor(1, 1)
robot.screen.print(f"Rotation: {robot.inertial.get_rotation():.2f}")
wait(50, MSEC)
reset_heading#
reset_heading
将机器人的航向重置为 0 度。
用法:
robot.inertial.reset_heading()
参数 |
描述 |
---|---|
该方法没有参数。 |
# Turn the robot around using a new 90 degree heading
robot.turn_to(90)
wait(1, SECONDS)
robot.inertial.reset_heading()
robot.turn_to(90)
set_heading#
set_heading
将机器人的航向设置为 0 到 359.99 度范围内的指定值。
用法:
robot.inertial.set_heading(航向)
参数 |
描述 |
---|---|
|
新航向使用的值在 0 至 359.99 度范围内。 |
# Turn the robot to 90 degrees using its new heading
robot.inertial.set_heading(45)
robot.turn_to(90)
碰撞#
crashed#
crashed
注册了一个当机器人检测到碰撞时要调用的函数。
用法:
robot.inertial.crashed(回调,arg)
参数 |
描述 |
---|---|
|
先前定义的在检测到碰撞时执行的 函数。 |
|
可选。包含要传递给回调函数的参数的元组。更多信息请参阅使用带参数的函数。 |
# Define what happens when a crash occurs
def crash_detected():
# Stop all movement and indicate a crash occurred
robot.screen.print("Crash detected")
robot.stop_all_movement()
robot.inertial.crashed(crash_detected)
# Drive forward until crash
robot.move_at(0, 100)
set_crash_sensitivity#
set_crash_sensitivity
调整触发碰撞响应所需的加速度阈值。
Usage:
robot.inertial.set_crash_sensitivity(sensitivity)
参数 |
描述 |
---|---|
|
碰撞敏感度:
<li>— 默认敏感度,1.5G 时触发。4</li> |
def crashed_callback():
robot.stop_all_movement()
robot.sound.play(CRASH)
# system event handlers
robot.inertial.crashed(crashed_callback)
# add 15ms delay to make sure events are registered correctly.
wait(15, MSEC)
# Detect a crash at a slow velocity.
robot.set_move_velocity(35, PERCENT)
robot.inertial.set_crash_sensitivity(SensitivityType.HIGH)
robot.move_at(0)
运动#
get_acceleration#
get_acceleration
以 -4.00 到 4.00 g 范围内的浮点数形式返回机器人在指定方向上的加速度。
用法:
robot.inertial.get_acceleration(类型)
参数 |
描述 |
---|---|
|
返回加速度的方向: 向下 - 影响机器人垂直运动的加速度。向前 - 影响机器人前后方向运动的加速度。向右 - 影响机器人左右方向运动的加速度。 |
# Display the acceleration as the robot begins to move
robot.screen.set_cursor(4,1)
sitting_accel = robot.inertial.get_acceleration(RIGHTWARD)
robot.screen.print(f"Resting: {sitting_accel:.2f}")
wait(0.5, SECONDS)
robot.screen.next_row()
robot.move_at(90, 100)
wait(0.1, SECONDS)
robot.screen.print(f"Startup: {robot.inertial.get_acceleration(RIGHTWARD):.2f}")
get_turn_rate#
get_turn_rate
以浮点数返回机器人的转弯速率(以每秒度数 (DPS) 为单位),范围从 -1000.00 到 1000.00 dps。
下图使用箭头显示滚动、俯仰和偏航的正旋转方向。
用法:
robot.inertial.get_turn_rate(轴)
参数 |
描述 |
---|---|
|
返回哪个方向: |
# Display the gyro rate as the robot is rotated by hand
while True:
robot.screen.clear_screen()
robot.screen.set_cursor(1, 1)
robot.screen.print(robot.inertial.get_turn_rate(YAW))
wait(50, MSEC)
校准#
校准是一个内部程序,用于测量并补偿 2 秒内的传感器噪声和漂移。在此期间,机器人必须保持完全静止(即,在稳定的表面上,不受任何外部运动的影响)。校准期间的移动会导致结果不准确。
VEX 机器人启动后会尝试自动校准,直到检测到无运动为止。但是,如果在启动过程中搬运或移动机器人,传感器可能无法正确校准或产生错误的校准结果。
如果您的项目高度依赖精确的航向,或者您需要一致且可重复的转弯,那么在代码开头调用 calibrate
会有所帮助。最好在校准期间在机器人屏幕上显示类似“校准…”的消息,然后在校准完成后将其更新为“校准完成”,以提醒您(以及任何其他使用机器人的人)机器人在此期间必须保持静止。
calibrate#
calibrate
用于校准陀螺仪。校准是一个内部程序,用于测量并补偿 2 秒内的传感器噪声和漂移。在此期间,机器人必须保持完全静止(即在稳定的表面上,且不受任何外部运动的影响)。校准期间的移动会导致结果不准确。
VEX 机器人启动后会尝试自动校准,直到检测到无运动为止。但是,如果在启动过程中搬运或移动机器人,传感器可能无法正确校准或产生错误的校准结果。
用法:
robot.inertial.calibrate()
参数 |
描述 |
---|---|
该方法没有参数。 |
# Calibrate the gryo before moving
robot.inertial.calibrate()
robot.screen.show_emoji(THINKING)
wait(2,SECONDS)
robot.screen.show_emoji(PROUD)
robot.move_for(50, 90)
is_calibrating#
is_calibrating
返回一个布尔值,指示陀螺仪是否正在校准。
真
——陀螺仪正在校准。False
——陀螺仪未校准。
用法:robot.inertial.is_calibrating()
参数 |
描述 |
---|---|
该方法没有参数。 |
# Move after the calibration is completed
robot.inertial.calibrate()
while robot.inertial.is_calibrating():
robot.screen.show_emoji(THINKING)
wait(50, MSEC)
robot.screen.show_emoji(PROUD)
robot.move_for(50, 90)