控制器#
介绍#
单摇杆控制器采用 4 键布局,并配备一个摇杆,摇杆既可用作模拟输入,又可用作可按压按钮。这些输入使机器人能够检测按钮按下和摇杆移动,从而实现交互式、灵敏的控制。以下列出了所有可用方法:
Getters – 读取按钮、操纵杆和连接状态。
.pressing – 返回指定按钮是否被按下。
.position – 返回操纵杆指定轴的位置。
is_connected – 返回控制器是否已连接。
get_battery_level – 以百分比形式返回控制器的电池电量。
回调——响应按钮或操纵杆输入变化。
吸气剂#
.pressing#
.pressing
返回一个整数,指示控制器上的特定按钮当前是否被按下。此方法必须在特定的按钮对象上调用,例如 button_up
(请参阅下面的按钮对象完整列表)。
1
-指定的按钮被按下。0
——指定的按钮未被按下。
用法:
五个可用按钮对象之一可与此方法一起使用,如下所示:
按钮 |
命令 |
---|---|
|
|
|
|
|
|
|
|
|
|
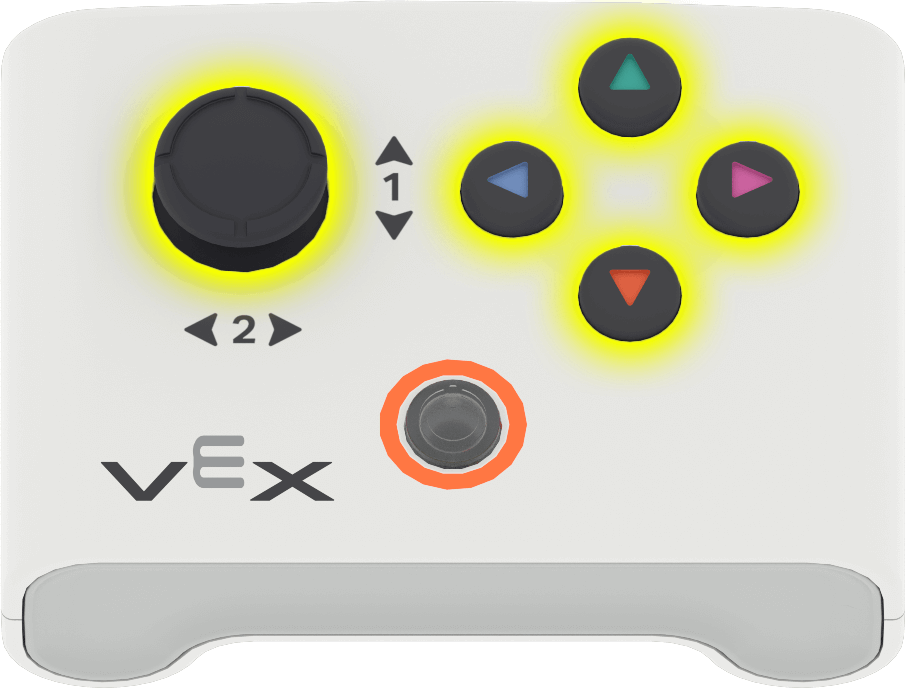
参数 |
描述 |
---|---|
该方法没有参数。 |
# Move forwards while the Up button is being pressed
while True:
if controller.button_up.pressing():
robot.move_at(0)
else:
robot.stop_all_movement()
.position#
.position
以 -100 到 100 之间的整数形式返回操纵杆指定轴的位置,表示百分比。
用法:
此方法可使用两个可用轴之一,如下所示:
按钮 |
命令 |
---|---|
|
|
|
|
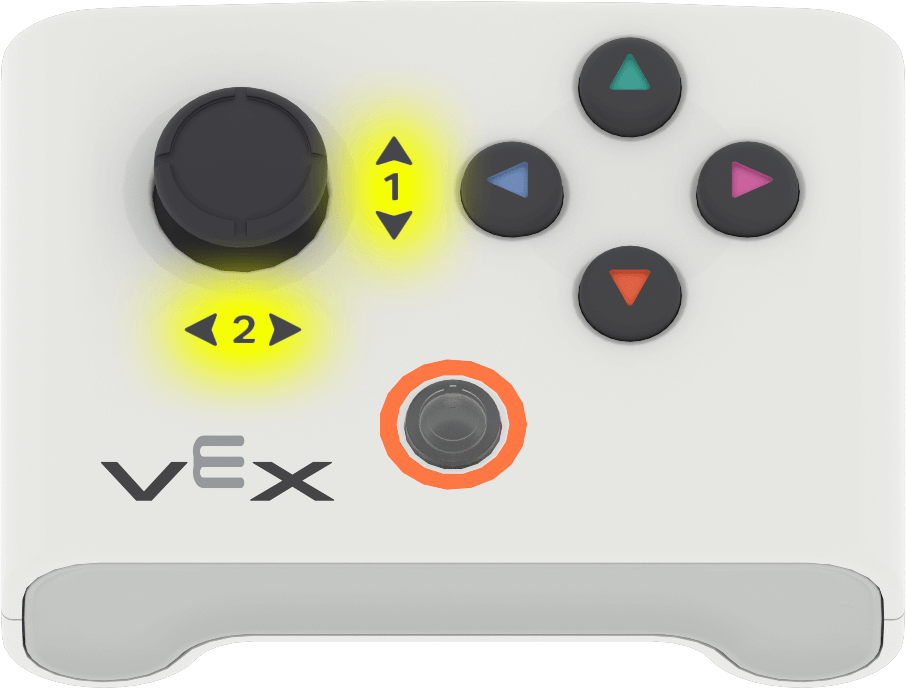
参数 |
描述 |
---|---|
该方法没有参数。 |
while True:
if controller.axis1.position() > 0:
# Move forward when the joystick is moved up
robot.move_at(0)
else:
# Stop moving when the joystick is centered
robot.stop_all_movement()
is_connected#
is_connected
返回一个布尔值,指示控制器是否已连接。
True
-控制器已连接。False
-控制器未连接。
用法:
controller.is_connected()
参数 |
描述 |
---|---|
该方法没有参数。 |
# Start moving forward.
# When the Controller disconnects, the robot stops.
robot.move_at(0)
while True:
if not controller.is_connected():
robot.stop_all_movement()
wait(100, MSEC)
get_battery_level#
get_battery_level
以 0 到 100 之间的整数形式返回控制器的电池电量,代表百分比。
用法:
controller.get_battery_level()
参数 |
描述 |
---|---|
该方法没有参数。 |
# Display the Controller's battery level.
robot.screen.print(controller.get_battery_level())
回调#
.pressed#
.pressed
注册一个函数,当控制器上的特定按钮被按下时调用。此方法必须在特定的按钮对象上调用,例如 button_up
–(请参阅下文按钮对象的完整列表)。
用法:
五个可用按钮对象之一可与此方法一起使用,如下所示:
按钮 |
命令 |
---|---|
|
|
|
|
|
|
|
|
|
|
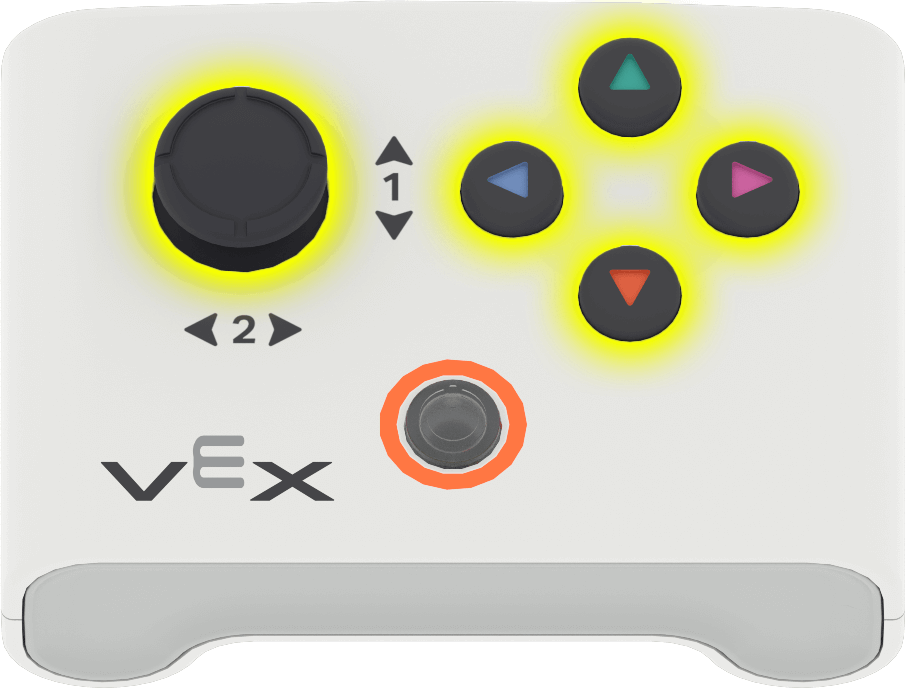
参数 |
描述 |
---|---|
打回来 |
先前定义的在按下指定按钮时执行的 函数。 |
arg |
可选。包含要传递给回调函数的参数的元组。更多信息请参阅使用带参数的事件。 |
# Kick hard when Up button is pressed
def kick_object():
robot.kicker.kick(HARD)
controller.button_up.pressed(kick_object)
.released#
.released
注册一个函数,当控制器上的特定按钮被释放时调用。此方法必须在特定的按钮对象上调用,例如 button_up
–(请参阅下文按钮对象的完整列表)。
用法:
五个可用按钮对象之一可与此方法一起使用,如下所示:
按钮 |
命令 |
---|---|
|
|
|
|
|
|
|
|
|
|
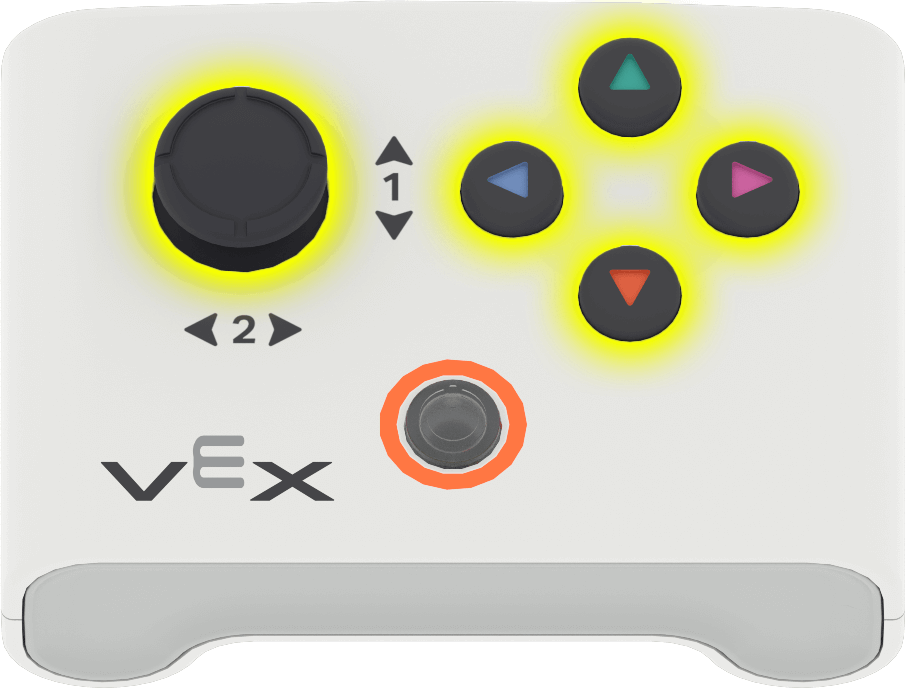
参数 |
描述 |
---|---|
打回来 |
先前定义的在释放指定按钮时执行的 函数。 |
arg |
可选。包含要传递给回调函数的参数的元组。更多信息请参阅使用带参数的事件。 |
# Clear the text after the Up button is released
def clear_screen():
robot.screen.clear_screen(BLUE)
controller.button_up.released(clear_screen)
robot.screen.print("Press Up, then")
robot.screen.next_row()
robot.screen.print("Release Up!")
.changed#
.changed
注册一个函数,当操纵杆的位置改变时调用。
用法:
此方法可以使用两个可用轴之一,如下所示:
按钮 |
命令 |
---|---|
|
|
|
|
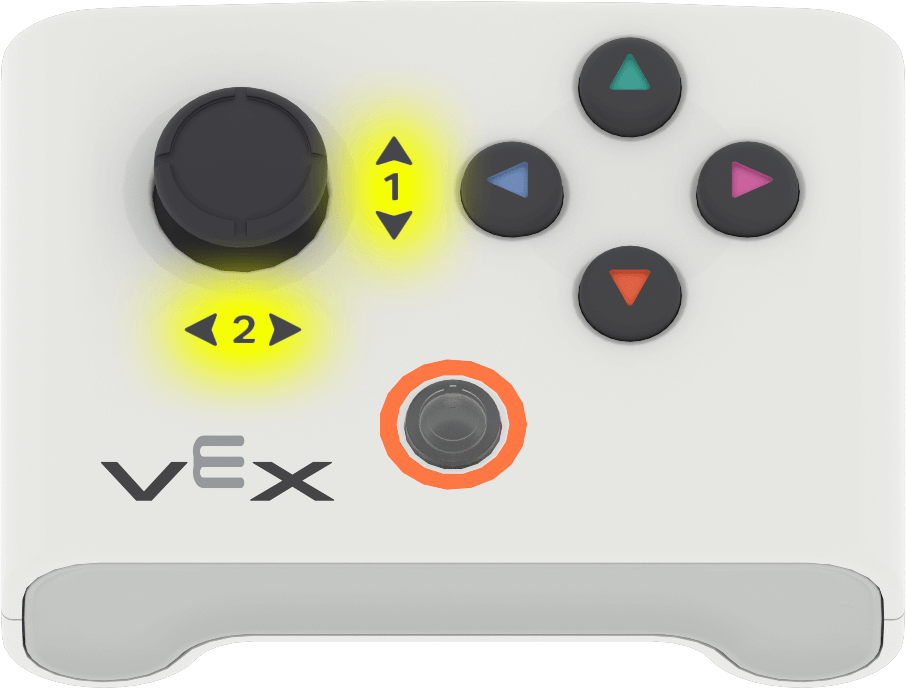
参数 |
描述 |
---|---|
打回来 |
先前定义的 函数,当轴的值发生变化时执行。 |
arg |
可选。包含要传递给回调函数的参数的元组。更多信息请参阅使用带参数的事件。 |
# Function to display an emoji when the joystick is moved
def move_joystick():
robot.screen.show_emoji(CONFUSED)
# Run the function when the joystick is moved up or down
controller.axis1.changed(move_joystick)