Inercial#
Introducción#
El sensor inercial del robot de codificación VEX AIM incluye un giroscopio de 3 ejes integrado para medir el movimiento de rotación y un acelerómetro de 3 ejes para detectar cambios de movimiento. Estos sensores permiten al robot rastrear su orientación, rumbo y aceleración. A continuación, se muestra una lista de todos los métodos disponibles:
Orientación: obtenga la dirección y los ángulos del robot.
get_rotation – Returns how much the robot has turned since it started.
get_heading – Returns the current heading (0 to 359.99°).
get_yaw – Returns the yaw angle (–180 to 180°).
get_roll – Returns the roll angle (–180 to 180°).
get_pitch – Returns the pitch angle (–90 to 90°).
reset_rotation – Resets the rotation value to zero.
reset_heading – Sets the current heading to zero.
set_heading – Sets the heading to a specific value.
Choque – Detecta colisiones.
crashed – Registers a function to run when a collision is detected.
Movimiento – Mide la aceleración y la velocidad de giro.
get_acceleration – Returns acceleration in a specific direction.
get_turn_rate – Returns turning rate in degrees per second.
Calibración – Administrar la calibración del sensor.
calibrate – Calibrates the inertial sensor.
is_calibrating – Returns whether the sensor is currently calibrating.
Orientación#
get_rotation#
get_rotation
devuelve la rotación neta del robot en grados como un flotante.
Uso:
robot.inertial.get_rotation()
Parámetros |
Descripción |
---|---|
Este método no tiene parámetros. |
# Display the robot's rotation as it rotates
while True:
robot.screen.clear_screen()
robot.screen.set_cursor(1, 1)
robot.screen.print(f"Rotation: {robot.inertial.get_rotation():.2f}")
wait(50, MSEC)
get_heading#
get_heading
devuelve el ángulo de rumbo del robot como un valor flotante en el rango de 0 a 359,99 grados.
Uso:
robot.inertial.get_heading()
Parámetros |
Descripción |
---|---|
Este método no tiene parámetros. |
# Turn right until the heading reaches 90 degrees
robot.turn(RIGHT)
while robot.inertial.get_heading() < 90:
wait(50, MSEC)
robot.stop_all_movement()
# Display the robot's heading as it is rotated by hand
while True:
robot.screen.clear_screen()
robot.screen.set_cursor(1, 1)
robot.screen.print(f"Heading: {robot.inertial.get_heading()} degrees")
wait(50, MSEC)
get_yaw#
get_yaw
devuelve el ángulo de guiñada del robot en el rango de –180,00 a 180,00 grados como un valor flotante.
La imagen a continuación utiliza flechas para mostrar la dirección de rotación positiva para guiñada.
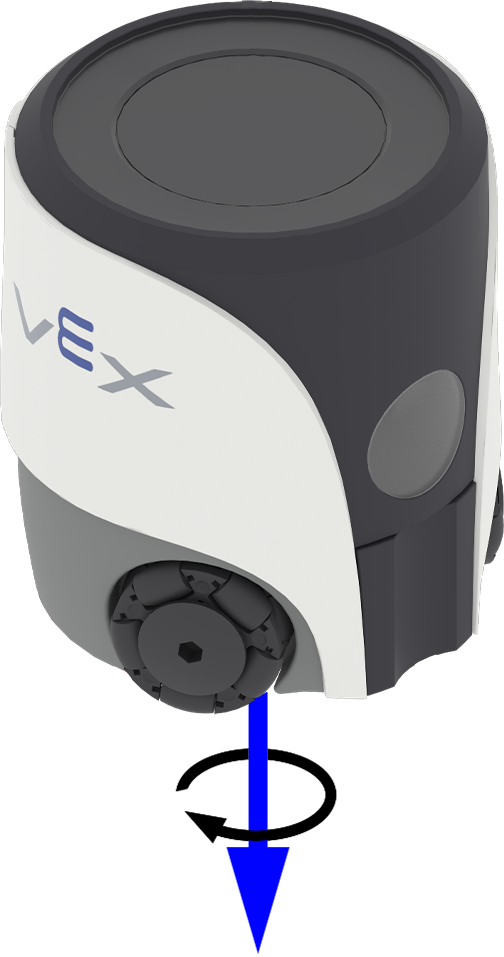
Uso:
robot.inertial.get_yaw()
Parámetros |
Descripción |
---|---|
Este método no tiene parámetros. |
# Display the robot's yaw angle as it is rotated by hand
while True:
robot.screen.clear_screen()
robot.screen.set_cursor(1, 1)
robot.screen.print(robot.inertial.get_yaw())
wait(50, MSEC)
get_roll#
get_roll
devuelve el ángulo de giro del robot en el rango de –180,00 a 180,00 grados como un valor flotante.
La imagen a continuación utiliza flechas para mostrar la dirección de rotación positiva del rollo.
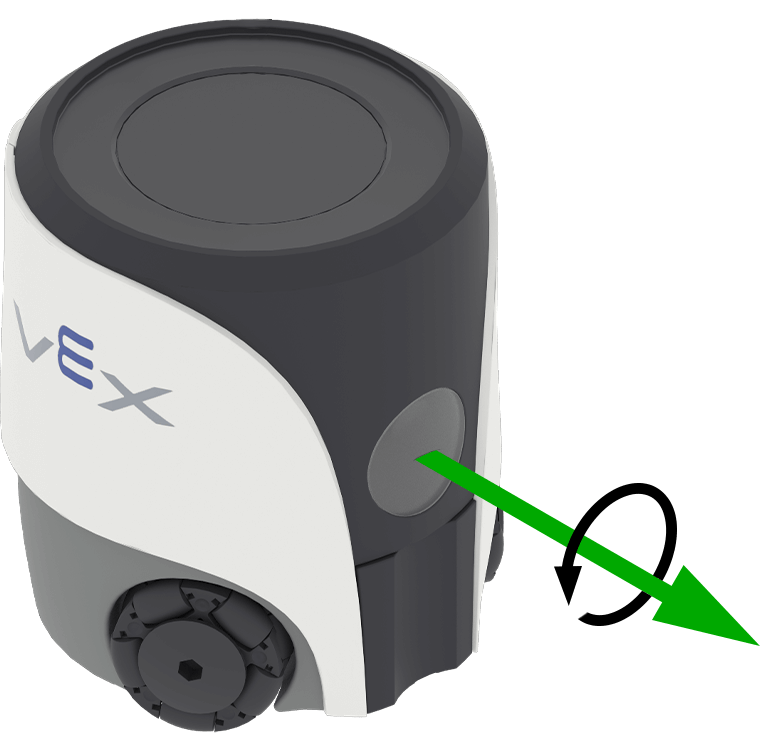
Uso:
robot.inertial.get_roll()
Parámetros |
Descripción |
---|---|
Este método no tiene parámetros. |
# Display the robot's roll angle as it is tilted by hand
while True:
robot.screen.clear_screen()
robot.screen.set_cursor(1, 1)
robot.screen.print(robot.inertial.get_roll())
wait(50, MSEC)
get_pitch#
get_pitch
devuelve el ángulo de inclinación del robot en el rango de –90,00 a 90,00 grados como un flotante.
La imagen a continuación utiliza flechas para mostrar la dirección de rotación positiva del paso.
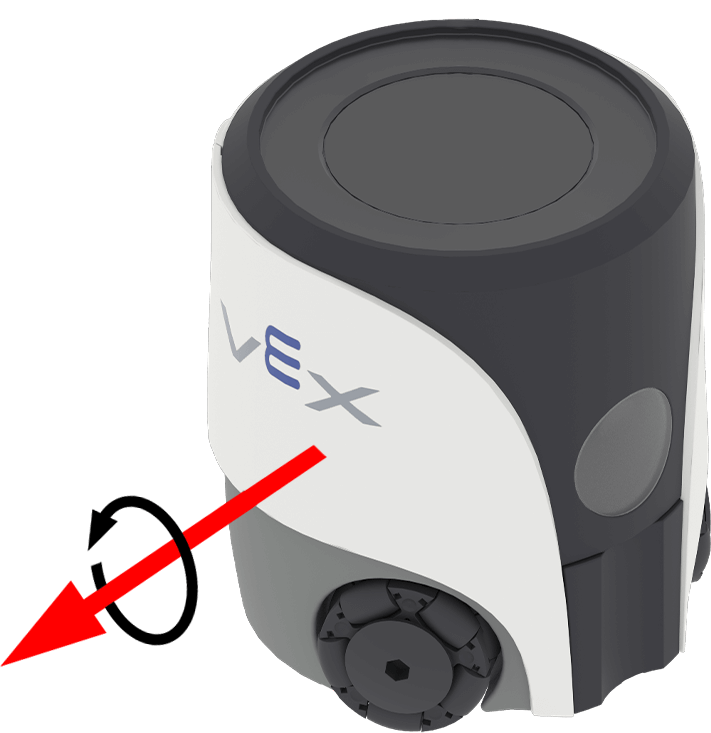
Uso:
robot.inertial.get_pitch()
Parámetros |
Descripción |
---|---|
Este método no tiene parámetros. |
# Display the robot's pitch angle as it is tilted by hand
while True:
robot.screen.clear_screen()
robot.screen.set_cursor(1, 1)
robot.screen.print(robot.inertial.get_pitch())
wait(50, MSEC)
reset_rotation#
reset_rotation
restablece el valor de rotación del giroscopio del robot a 0 grados.
Uso:
robot.inertial.reset_rotation()
Parámetros |
Descripción |
---|---|
Este método no tiene parámetros. |
# Reset the robot's rotation if it exceeds 180 degrees
while True:
if robot.inertial.get_rotation() >= 180:
robot.inertial.reset_rotation()
robot.screen.clear_screen()
robot.screen.set_cursor(1, 1)
robot.screen.print(f"Rotation: {robot.inertial.get_rotation():.2f}")
wait(50, MSEC)
reset_heading#
reset_heading
restablece el rumbo del robot a 0 grados.
Uso:
robot.inertial.reset_heading()
Parámetros |
Descripción |
---|---|
Este método no tiene parámetros. |
# Turn the robot around using a new 90 degree heading
robot.turn_to(90)
wait(1, SECONDS)
robot.inertial.reset_heading()
robot.turn_to(90)
set_heading#
set_heading
establece el rumbo del robot en un valor específico en el rango de 0 a 359,99 grados.
Uso:
robot.inertial.set_heading(heading)
Parámetros |
Descripción |
---|---|
|
El valor a utilizar para el nuevo rumbo en el rango de 0 a 359,99 grados. |
# Turn the robot to 90 degrees using its new heading
robot.inertial.set_heading(45)
robot.turn_to(90)
Chocar#
crashed#
crashed
registra una función que se llamará cuando el robot detecte una colisión.
Uso:
robot.inertial.crashed(callback, arg)
Parámetros |
Descripción |
---|---|
|
A function that is previously defined to execute when a collision is detected. |
|
Optional. A tuple containing arguments to pass to the callback function. See Using Functions with Parameters for more information. |
# Define what happens when a crash occurs
def crash_detected():
# Stop all movement and indicate a crash occurred
robot.screen.print("Crash detected")
robot.stop_all_movement()
robot.inertial.crashed(crash_detected)
# Drive forward until crash
robot.move_at(0, 100)
set_crash_sensitivity#
set_crash_sensitivity
ajusta el umbral de aceleración necesario para activar una respuesta de choque.
Uso:
robot.set_crash_sensitivity(sensitivity)
Parámetros |
Descripción |
---|---|
|
The crash sensitivity:
|
def crashed_callback():
robot.stop_all_movement()
robot.sound.play(CRASH)
# system event handlers
robot.inertial.crashed(crashed_callback)
# add 15ms delay to make sure events are registered correctly.
wait(15, MSEC)
# Detect a crash at a slow velocity.
robot.set_move_velocity(35, PERCENT)
robot.inertial.set_crash_sensitivity(SensitivityType.HIGH)
robot.move_at(0)
Movimiento#
get_acceleration#
get_acceleration
devuelve la aceleración del robot en una dirección especificada como un valor flotante en el rango de –4,00 a 4,00 g.
Uso:
robot.inertial.get_acceleration(type)
Parámetros |
Descripción |
---|---|
|
La dirección de aceleración para regresar: DOWNWARD - Aceleración que afecta el movimiento vertical del robot.FORWARD - Aceleración que afecta el movimiento del robot a lo largo de su dirección de adelante hacia atrás.RIGHTWARD - Aceleración que afecta el movimiento del robot a lo largo de su dirección de lado a lado. |
# Display the acceleration as the robot begins to move
robot.screen.set_cursor(4,1)
sitting_accel = robot.inertial.get_acceleration(RIGHTWARD)
robot.screen.print(f"Resting: {sitting_accel:.2f}")
wait(0.5, SECONDS)
robot.screen.next_row()
robot.move_at(90, 100)
wait(0.1, SECONDS)
robot.screen.print(f"Startup: {robot.inertial.get_acceleration(RIGHTWARD):.2f}")
get_turn_rate#
get_turn_rate
devuelve la velocidad de giro del robot en grados por segundo (DPS) como un flotante, de –1000,00 a 1000,00 dps.
La imagen a continuación utiliza flechas para mostrar la dirección de rotación positiva para balanceo, cabeceo y guiñada.
Uso:
robot.inertial.get_turn_rate(axis)
Parámetros |
Descripción |
---|---|
|
¿Qué orientación devolver: |
# Display the gyro rate as the robot is rotated by hand
while True:
robot.screen.clear_screen()
robot.screen.set_cursor(1, 1)
robot.screen.print(robot.inertial.get_turn_rate(YAW))
wait(50, MSEC)
Calibración#
La calibración es un procedimiento interno que mide y compensa el ruido y la deriva del sensor durante un periodo de 2 segundos. Durante este tiempo, el robot debe permanecer completamente inmóvil (es decir, sobre una superficie estable sin ningún movimiento externo). El movimiento durante la calibración producirá resultados inexactos.
Los robots VEX intentan calibrarse automáticamente al iniciarse, esperando hasta que no detecten movimiento. Sin embargo, si el robot se transporta o se mueve durante el inicio, el sensor podría no calibrarse correctamente o generar una calibración incorrecta.
Si su proyecto depende en gran medida de un rumbo preciso, o si necesita giros consistentes y repetibles, llamar a calibrate
al principio del código puede ser útil. Es recomendable mostrar un mensaje como “Calibrando…” en la pantalla del robot durante la calibración y luego actualizarlo a “Calibración completada” para recordarle a usted (y a cualquier otra persona que use el robot) que el robot debe permanecer inmóvil durante este periodo.
calibrate#
calibrate
calibra el giroscopio. La calibración es un procedimiento interno que mide y compensa el ruido del sensor y la deriva durante un período de 2 segundos. Durante este tiempo, el robot debe permanecer completamente inmóvil (es decir, sobre una superficie estable sin ningún movimiento externo). El movimiento durante la calibración producirá resultados inexactos.
Los robots VEX intentan calibrarse automáticamente al iniciarse, esperando hasta que no detecten movimiento. Sin embargo, si el robot se transporta o se mueve durante el inicio, el sensor podría no calibrarse correctamente o generar una calibración incorrecta.
Uso:
robot.inertial.calibrate()
Parámetros |
Descripción |
---|---|
Este método no tiene parámetros. |
# Calibrate the gryo before moving
robot.inertial.calibrate()
robot.screen.show_emoji(THINKING)
wait(2,SECONDS)
robot.screen.show_emoji(PROUD)
robot.move_for(50, 90)
is_calibrating#
is_calibrating
devuelve un valor booleano que indica si el giroscopio está calibrando.
True
- El giroscopio se está calibrando.False
: El giroscopio no está calibrando.
Uso: robot.inertial.is_calibrating()
Parámetros |
Descripción |
---|---|
Este método no tiene parámetros. |
# Move after the calibration is completed
robot.inertial.calibrate()
while robot.inertial.is_calibrating():
robot.screen.show_emoji(THINKING)
wait(50, MSEC)
robot.screen.show_emoji(PROUD)
robot.move_for(50, 90)